How to check whether a string contains a substring in JavaScript?
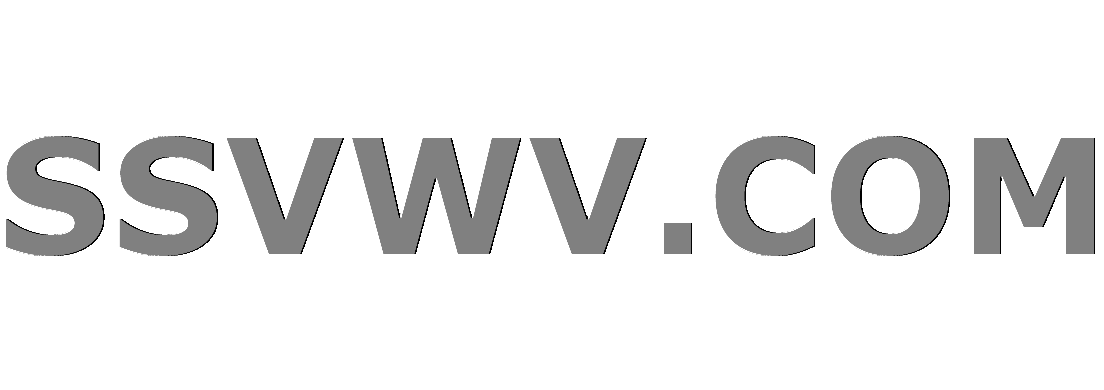
Multi tool use
Usually I would expect a String.contains()
method, but there doesn't seem to be one.
What is a reasonable way to check for this?
This question's answers are a collaborative effort: if you see something that can be improved, just edit the answer to improve it! No additional answers can be added here
Here is a list of current possibilities:
1. (ES6) includes
—go to answer
2. ES5 and older indexOf
String.prototype.indexOf
returns the position of the string in the other string. If not found, it will return -1
.
3. search
—go to answer
4. lodash includes—go to answer
5. RegExp—go to answer
6. Match—go to answer
Performance tests are showing that indexOf
might be the best choice, if it comes to a point where speed matters.
You can easily add a contains
method to String with this statement:
Note: see the comments below for a valid argument for not using this. My advice: use your own judgement.
Alternatively:
The problem with your code is that JavaScript is case sensitive. Your method call
should actually be
Try fixing it and see if that helps:
There is a string.includes
in ES6:
Note you may need to load es6-shim
or similar to get this working on older browsers.
You could use the JavaScript search()
method.
Syntax is: string.search(regexp)
It returns the position of the match, or -1 if no match is found.
See examples there: jsref_search
You don't need a complicated regular expression syntax. If you are not familiar with them a simple st.search("title")
will do. If you want your test to be case insensitive, then you should do st.search(/title/i)
.
String.prototype.includes()
was introduced in ES6.
Determines whether one string may be found within another string,
returning true or false as appropriate.
A string to be searched for within this string.
The position in this string at which to begin searching for searchString
defaults to 0.
This may require ES6 shim in older browsers.
If you were looking for an alternative to write the ugly -1 check, you prepend a ~ tilde instead.
Joe Zimmerman - you'll see that using ~ on -1 converts it to 0. The number 0 is a
falsey value, meaning that it will evaluate to false when converted to
a Boolean. That might not seem like a big insight at first, but
remember functions like indexOf will return -1 when the query is not
found. This means that instead of writing something similar to this:
You can now have fewer characters in your code so you can write it
like this:
More details here
This piece of code should work well:
A common way to write a contains
method in JavaScript is:
The bitwise negation operator (~
) is used to turn -1
into 0
(falsey), and all other values will be non-zero (truthy).
The double boolean negation operators are used to cast the number into a boolean.
In ES5
In ES6 there are three new methods: includes()
, startsWith()
, endsWith()
.
Instead of using code snippets found here and there on the web, you can also use a well-tested and documented library. Two Options I would recommend:
1st option: Use Lodash: It has an includes
method:
Lodash is the most popular javascript library dependency for npm and has loads of handy javascript utility methods. So for many projects you would want this anyway ;-)
2nd option: Or use Underscore.string: It has an include
method:
Here is the description of Underscore.string, it just adds 9kb but gives you all the advantages a well-tested and documented library has over copy'n'paste code snippets:
Underscore.string is JavaScript library for comfortable manipulation
with strings, extension for Underscore.js inspired by Prototype.js,
Right.js, Underscore and beautiful Ruby language.
Underscore.string provides you several useful functions: capitalize,
clean, includes, count, escapeHTML, unescapeHTML, insert, splice,
startsWith, endsWith, titleize, trim, truncate and so on.
Note well, Underscore.string is influenced by Underscore.js but can be used without it.
Last not Least: With JavaScript version ES6 comes an built-in includes
method:
Most modern browsers already support it, have an eye on the ES6 compatibility table.
You can use jQuery's :contains
selector.
Check it here: contains-selector
Use a regular expression:
RegExp.test(string)
This just worked for me. It selects for strings that do not contain the term "Deleted:"
You were looking for .indexOf
MDN.
indexOf
is going to return an index to the matched substring. The index will correlate to where the substring starts. If there is no match, a -1 is returned. Here is a simple demo of that concept:
Another option of doing this is:
You can use the match function, that is, something like:
match() can also work with regular expression :
You need to call indexOf with a capital "O" as mentioned. It should also be noted, that in JavaScript class is a reserved word, you need to use className to get this data attribute. The reason it's probably failing is because it's returning a null value. You can do the following to get your class value...
Since the question is pretty popular, I thought I could add a little modern flavor to the code.
BTW, the correct answer is misspelling indexOf
or the non-standard String.contains
.
Loading an external library (especially if the code is written in pure JavaScript) or messing with String.prototype
or using a regular expression is a little overkill.
There is a sleek and better way to do this and it is using the (BitWise NOT) operator.
The Bitwise Not converts "x" into -(x + 1) so, if the x turns out -1 from indexOf method.then it will be converted into -( -1 + 1) = -0 which is a falsy value .
As others have already mentioned, JavaScript strings have both an indexOf
and search
method.
The key difference between both, is that indexOf
is for plain substrings only, whereas search
also supports regular expressions. Of course, an upside of using indexOf
is that it's faster.
See also In JavaScript, what is the difference between indexOf() and search()?.
If you want to add your own contains
method to every string, the best way to do it would be @zzzzBov's approach:
You would use it like this:
It is generally frowned upon to add your own custom methods to standard objects in JavaScript, for example, because it might break forward compatibility.
If you really want your own contains
method and/or other custom string methods, it's better to create your own utility library and add your custom string methods to that library:
You would use it like this:
If you don't want to create your own custom helper library, there is - of course - always the option of using a third-party utility library. As mentioned by @nachtigall, the most popular ones are Lodash and Underscore.js.
In Lodash, you could use _.includes()
, which you use like this:
In Underscore.js, you could use _.str.include()
, which you use like this :
Simple workaround
you can use in the following way
MDN reference
Example
JavaScript code to use the contains
method in an array:
In the given code the contains
method determines whether the specified element is present in the array or not. If the specified element is present in the array, it returns true, otherwise it returns false.
ES6 contains String.prototype.includes
.
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/String/includes
To collect some kind of valid solutions:
Since there is a complaint about using the prototype, and since using indexOf
makes your code less readable, and since regexp is overkill:
That is the compromise I ended up going for.
JavaScript
jQuery
Use the inbuilt and simplest one i.e match()
on the string. To achieve what you are looking forward do this:
The easyest way is indeed using indexOf. To just check a string string
for a substring substr
you can use this method:
As you wanted the function string.contains()
, you can implement it yourself like this:
Now you can use this ecen shorter method to check if a string contains a special substring:
Here is a JSFiddle as well.
Thank you for your interest in this question.
Because it has attracted low-quality or spam answers that had to be removed, posting an answer now requires 10 reputation on this site (the association bonus does not count).
Would you like to answer one of these unanswered questions instead?